This function produces a single cumulative value by running a Child Flow for each item in a list. For example you can determine the largest number in a list of numbers, count how many text items start with “A” in a list of text, etc. The result can be any type – not just a number – so you can also use Reduce to build text, date, true/false, object, or a list of any of those.
Basically this works by calling a Flow on each item in the list, which outputs a “memo” value. This memo is passed as input to the next iteration, and so on until the whole list has been processed - at that point, the final memo value becomes the output of this card.
To use this function, you’ll need to create the Child Flow that gets called for each item. Define fields on the event card that will be the inputs that determine how to increment the cumulative value. At a minimum, you’ll need a field for the item from the list (which you can name whatever you want) and a field that will keep track of the accumulated value (which must be named “memo”).
You can also include other inputs that your Flow may need. After defining your inputs, build the rest of the child Flow, ending with a Return card (found in the Control category) that returns the new accumulated value – to be passed along with the next item in the list to the next call of the child Flow, or if there are no more items in the list, this will be the result of the Reduce function. If the accumulated value is a type other than an object (e.g. a number), there should be a single return field of that type and its name doesn’t matter. If the accumulator is an object, then there should be one field for each of the keys of the object and the names of the fields must match the key names.
Fill out the Reduce card as follows:
- list: The list to operate on
- flow: The child Flow (as described above) that will be called once for each item in the list
- with the following values (dynamically generated):
- item: (will have whatever name you chose when you created your child Flow) Click in the field that will hold the current item from the list and choose “Item.” (Or, in some instances, you may also be able to select a specific path within an object.)
- memo: Set the type of this field to be the type you want your final result to be (e.g. if you’re using Reduce to get a sum, then the type should be number). Provide the starting value (e.g. for doing a sum, you’ll want to start with zero.)
- You can have optional additional fields on the child Flow event card in child Flow you chose, and those will also show up as input fields.
Output Fields
- item: The resulting value after the child Flow has executed on each of the items. Make sure to set the type of item to match the type of the input memo.
Examples
Let’s say you have a list of numbers and want to use Reduce to determine the largest of those numbers. First you’ll need to create a child Flow. Start the Flow with a child Flow event and add two fields: “current item” (a number) and “memo” (a number).
The next card is a Control - AssignIf function that outputs the larger of those two numbers.
The final card is a Control - Return function that returns the output from the AssignIf card as the new memo. Save that Flow as “inner - max” and turn it on.
Now, you can create a List Reduce card that accepts a list of number. Click the “Choose Flow” button and select the “inner-max” Flow you just created. Click the “current item” input and select “Item” from the dropdown. Click the “memo” input and enter zero (as the start value).
Here’s what your Reduce card will look like:
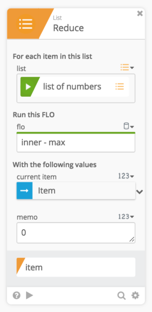
Here’s what the child Flow looks like:
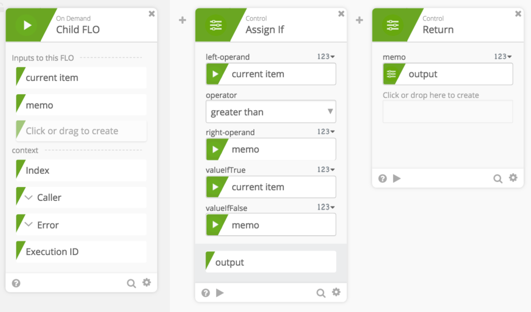
Here’s how the Reduce card will work for the above example:
Let’s say you passed it a list value of [1, 7, 4, 19, 2]
. The “inner-max” child Flow will get called 5 times – once for each item in the list as follows:
- The first time, current item is 1 and memo is 0. The child Flow returns the larger of the two: 1.
- The next time, current item is 7 and memo is 1 (the return value from the previous step). The child Flow compares them and returns 7.
- The third time, current item is 4 and memo is 7. The child Flow returns 7.
- The fourth time, current item is 19 and memo is 7. The child Flow returns 19.
- The final time, current item is 2 and memo is 19. The child Flow returns 19, and since there are no more items in the list, this becomes the final output.
The above example involves simple math, but you can make your child Flow as complex as you need.
It can contain If/Else conditions, complex calculations, or application actions.
And it’s not limited to numbers and math: it can build a different type, such as text (e.g. using Concatenate) or a list (e.g. using List Add to End).
Just make sure that the types match for memo and item on the Reduce card and the memo field on the child Flow’s event card. And the value returned by the child Flow on the Return card must also match unless the type is object, in which case there must be a field on the Return card that corresponds to each key of the object and they are named the same as the keys.