This workbook is designed to accompany section 206 - JavaScript and Call Code Function Card.
Pre-Requisites
For this workbook, you should have your own environment with login credentials.
If you are using a Production environment, please take care with any existing processes.
You may also elect to use 30-day free Trial Org by visiting: https://azuqua.com/sign-up
Because this is an Integration product, we will encourage you to use freely-available applications within this material. Tools such as Twitter, Slack, and others are great products and typically allow you to sign-up for free!
Exercise 206-1 - A Simple Average Function
Let’s create a very simple Flow and test our function!
- As usual, click +New Flow
- Let’s name this “Call Code 1 - Average” and give it a description
- To begin, let’s assign two numbers to calculate using “Add” Card
- Click the + to make a Call Code Function.
- Select the Average Function (and Average for the function within it)
- Set your count to “2” (you have two values)
- And let’s create a very simple “Compose” card that will give us the result.
To test, set easy values of 4 and 2, then run the Flow. You should receive a response of “3” meaning that it works!
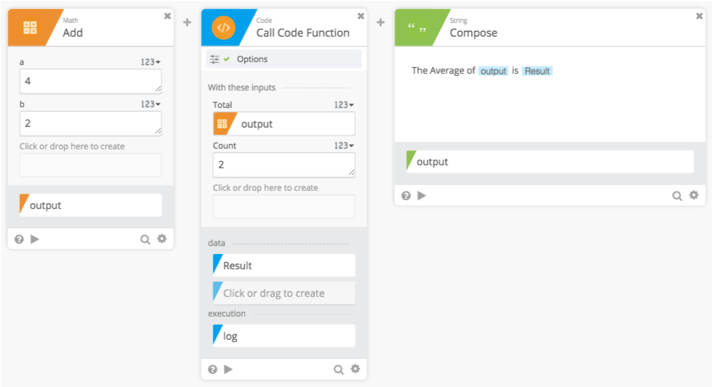
Exercise 206-2 - Calculating a Percent Difference
Let’s now work another example that typifies working with “found code”. This implies that someone else has crafted a useful JavaScript function that you want to use - and you’ll have to make changes.
Let’s calculate Percent Difference between two values. This is defined as follows:
Percentage change : 100 % times the (Fractional change )
After an internet search, a nice piece of JavaScript is herehere:
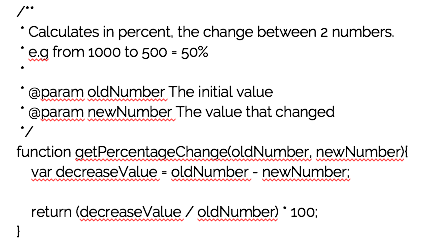
Try loading this into the code section and see what happens! Hint: You can expect errors (which we’ll fix in the next section).
Exercise 206-3 - Fixing the Percent Difference Code
We can, however, get this to work.
First, let’s start with the Comment Block. Remember, this is CRUCIAL because it outlines the values (variables) we want to pass back and forth. We need to make some changes and include a name
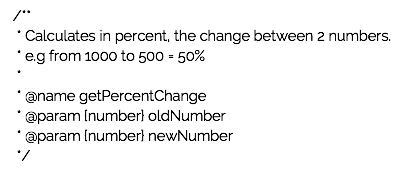
This isn’t changed - much - but now we also are TYPING the parameters. That is what tells our Flow what kind of variable we’re working with (JavaScript is not strongly typed).
Exercise 206-4 - Fixing the Body of the Code
Now we have to spin this to work.
Remember we have the “req” and “res” parameters to work with … so we need to change the code within the body so it will work correctly!
To summarize the work you’ll need to do (see the image following for exact details):
- Change the function name (to getPercentageChange(req,res))
- Extract the input parameters (Var inputs = req.body.data;)
- Catch errors (Divide by Zero)
- Do the math!
- Return the result (res.status(200).send({pct_difference”:output”});)
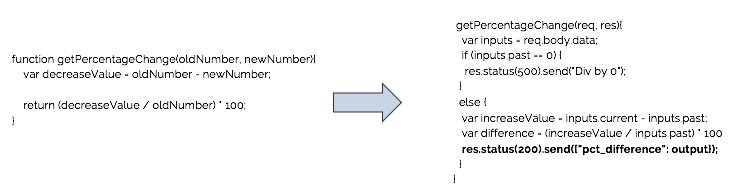
Exercise 206-5 Testing and Troubleshooting
So let’s save and set up the card …
- Call Code Card
- Select the code I want
- Configure it
- Test the card
Upon execution, you should see that you have a problem here … there’s no RESULT!
So let’s switch back to the code and see what we missed … You should find that you’re missing the RETURN parameter.
Within the comments block, add the following line (omit the first bullet!):
- * @return {number} pct_difference
Running it Again:
And now we see that we do get a result, however, that result is not quite in the format we want. I really want to return a value with a certain number of decimal places … (like only 1).
Consulting JavaScript documentation, I see that the .toFixed(n) option will do what I want, so let’s add this line:
var output = difference.toFixed(1);
If you re-run, this should work as designed!